Elevate Your Coding: 10 Essential C# .NET Snippets
Written on
Chapter 1: The Significance of C# and .NET
In the fast-paced realm of software development, C# and the .NET Framework serve as foundational elements for creating sturdy and scalable applications. Their rich feature set and user-friendly syntax can significantly transform your projects. This article highlights 10 essential snippets designed to elevate your coding abilities, allowing you to appreciate the elegance of streamlined and effective code.
Section 1.1: Read-Only Collections
Immutable collections are crucial for thread safety and maintaining data integrity.
var originalList = new List<string> { "Alice", "Bob", "Charlie" };
var readOnlyCollection = originalList.AsReadOnly();
Now, readOnlyCollection is immutable.
Section 1.2: Async/Await for Enhanced Responsiveness
Utilize async/await to ensure your user interface remains responsive while operations proceed asynchronously.
public async Task<string> FetchDataAsync(string url)
{
using (var httpClient = new HttpClient())
{
var response = await httpClient.GetStringAsync(url);
return response;
}
}
Chapter 2: Powerful Data Manipulation with LINQ
Section 2.1: LINQ Queries
Easily handle data manipulation using LINQ queries to improve code readability.
var scores = new int[] { 97, 92, 81, 60 };
var highScores = from score in scores
where score > 80
select score;
highScores now contains 97, 92, and 81.
Section 2.2: Null-Conditional Operator
Prevent NullReferenceException by employing the null-conditional operator for safer checks.
string[] array = null;
var length = array?.Length ?? 0;
Here, length equals 0 without causing an exception.
Section 2.3: Tuple Deconstruction
Simplify method returns by utilizing tuples and deconstruction.
public (int, string) GetPerson()
{
return (1, "John Doe");
}
var (id, name) = GetPerson();
Section 2.4: ValueTuple for Lightweight Structures
Make use of ValueTuple for temporary data storage without needing a full class or struct.
var person = (Id: 1, Name: "Jane Doe");
Console.WriteLine($"{person.Name} has ID {person.Id}");
Section 2.5: Embracing Pattern Matching
Adopt pattern matching for clearer syntax when verifying types and values.
object obj = 123;
if (obj is int i)
{
Console.WriteLine($"Integer: {i}");
}
Section 2.6: Extension Methods
Augment the capabilities of existing classes without changing their source code.
public static class StringExtensions
{
public static string Quote(this string str)
{
return $""{str}"";}
}
var myString = "Hello, world!";
Console.WriteLine(myString.Quote());
Section 2.7: Using Declarations
Facilitate the management of disposable objects through the new using declaration.
using var streamReader = new StreamReader("file.txt");
string content = streamReader.ReadToEnd();
The StreamReader is automatically disposed of in this context.
Section 2.8: Dynamic LINQ to SQL
Dynamic LINQ allows for adaptable database queries as your application's requirements change.
using (var context = new DataContext())
{
var query = context.People.Where("City == @0 and Age > @1", "Seattle", 25);
foreach (var person in query)
{
Console.WriteLine(person.Name);}
}
By incorporating these snippets into your regular coding practices, you can enhance both the efficiency and clarity of your code, while fully harnessing the capabilities of C# and .NET to create impactful and scalable applications. These snippets serve as a toolkit for exploring the expansive domain of .NET programming, ensuring that every line you write demonstrates your commitment to quality and professionalism.
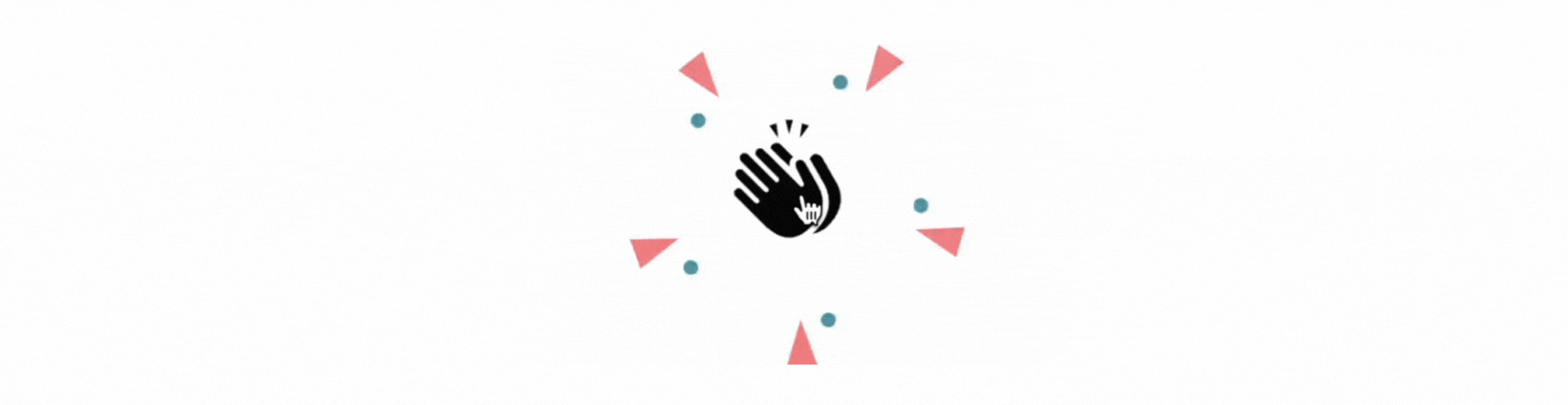