Optimize Web App Performance with JavaScript Event Delegation
Written on
Chapter 1: Introduction to Event Delegation
As web applications become more intricate, the need for performance optimization is crucial for ensuring seamless user interactions. One of the most effective methods to achieve this is through event delegation, which reduces memory usage while significantly improving rendering speeds.
In this section, we will explore the benefits of implementing event delegation frameworks, supported by practical examples.
Prerequisites
Before diving into the mechanics of event delegation, readers should have an intermediate understanding of HTML, CSS, and ES6+ JavaScript. For foundational concepts, consider referencing resources like Mozilla Developer Network (MDN), freeCodeCamp, or Coursera courses.
Section 1.1: Traditional Event Handling vs. Event Delegation
Traditional methods often require assigning separate event listeners to each element, leading to substantial overhead and increased resource consumption. In contrast, event delegation involves attaching a single event handler to a common ancestor, which manages the events of its child elements. This approach saves computational resources and enhances performance.
Subsection 1.1.1: Implementation Techniques
To implement delegated event listeners, use the addEventListener() method with the appropriate capture flags set to true. This allows for lower-level event capturing, enabling precise identification of events as they bubble up through the DOM hierarchy.
Extract the source of the event using event.target to pinpoint the specific child element that triggered the event.
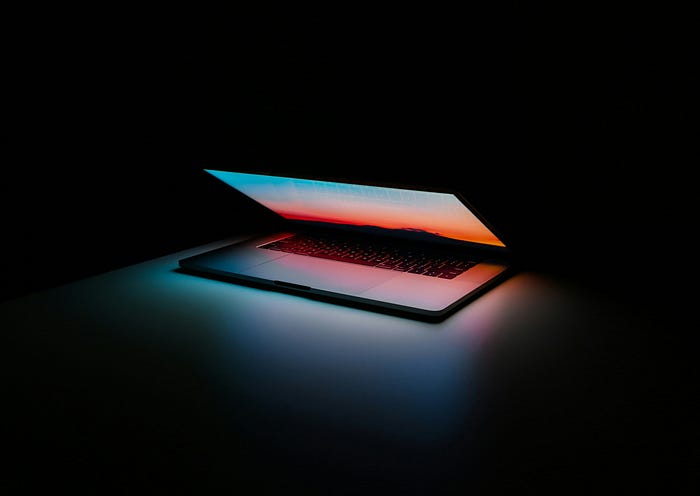
Section 1.2: Practical Example
Consider a scenario where you have dynamically generated lists that require a delete functionality without cluttering your markup with inline scripts. By using event delegation, you can maintain clean separation of concerns and improve maintainability.
HTML Template:
Add Entry
JavaScript Logic:
const listContainer = document.querySelector("#list");
listContainer.addEventListener(
"click",
(event) => {
// Ensure the target matches the intended criteria (li elements)
if (!event.target.matches("li")) return;
// Perform delete operation
event.target.remove();},
true
);
function addEntry() {
const entryTemplate = <li>New Item</li>;
listContainer.insertAdjacentHTML("beforeend", entryTemplate);
}
Chapter 2: Benefits of Event Delegation
Event delegation provides various advantages, including:
- Decreased memory usage
- Enhanced rendering times
- Improved maintainability
- Greater ease of extensibility
Best Practices:
- Position delegate listeners high enough in the DOM to maintain control while conserving resources.
- Be cautious about isolating genuine events and filtering out unnecessary noise.
- Regularly test for cross-browser compatibility to ensure consistent functionality.
The first video, "Event Delegation in JavaScript Explained in 5 Minutes," succinctly demonstrates the principles of event delegation and its impact on performance.
The second video, "Event Delegation in JavaScript, Simplified," breaks down the concept into easy-to-understand segments, making it accessible for all developers.
Conclusion
Adopting event delegation can significantly enhance efficiency, leading to improved overall quality in web applications. By combining effective debugging practices, thorough benchmark testing, and routine refactoring, developers can achieve measurable performance improvements, benefiting end-users immensely.