Effortless Form Management in React Applications Using Formik
Written on
Chapter 1: Introduction to Formik
Formik is a powerful library designed to streamline form handling in React applications. In this discussion, we will explore how to manage form inputs effectively using Formik, with a special focus on field-level validation.
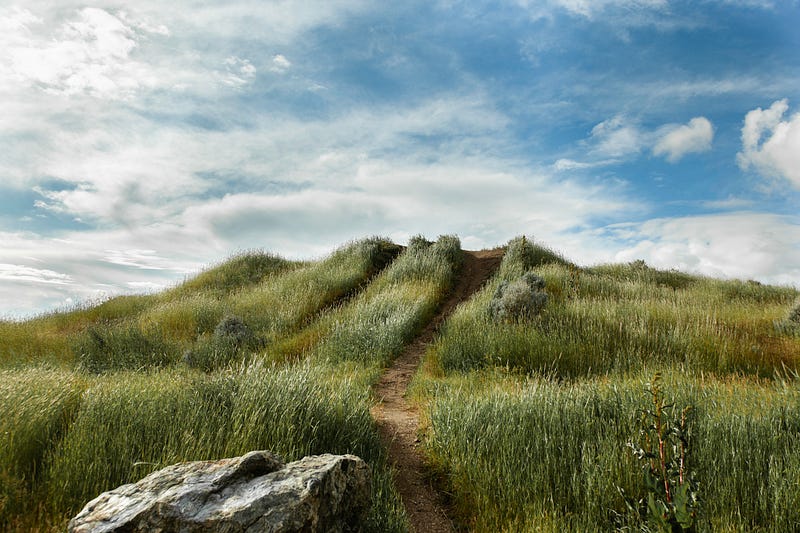
Field-Level Validation in Formik
To implement field-level validation, we create custom validation functions and pass them to the validate property of the Field component. Here’s a simple example:
import React from "react";
import { Formik, Form, Field } from "formik";
function validateEmail(value) {
let error;
if (!value) {
error = "This field is required";} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+.[A-Z]{2,4}$/i.test(value)) {
error = "Please enter a valid email address";}
return error;
}
export const FormExample = () => (
<div>
<h1>Sign Up</h1>
<Formik
initialValues={{ email: "" }}
onSubmit={(values) => {
console.log(values);}}
>
{({ errors, touched }) => (
<Form>
<Field name="email" validate={validateEmail} />
{errors.email && touched.email && <div>{errors.email}</div>}
<button type="submit">Submit</button>
</Form>
)}
</Formik>
</div>
);
In this code, we define the validateEmail function to check the email input. If the value is invalid, an appropriate error message is returned. Within the FormExample, we set up the form with Formik and Form components. The initialValues property holds the starting value for each field, and the Field component uses the same name prop as defined in initialValues.
Manually Triggering Validation
Formik also allows for manual validation triggers. Below is an example demonstrating how to implement this feature:
import React from "react";
import { Formik, Form, Field } from "formik";
function validateUsername(value) {
let error;
if (value === "admin") {
error = "Nice try!";}
return error;
}
export const FormExample = () => (
<div>
<h1>Sign Up</h1>
<Formik
initialValues={{ username: "" }}
onSubmit={(values) => {
console.log(values);}}
>
{({ errors, touched, validateField, validateForm }) => (
<Form>
<Field name="username" validate={validateUsername} />
{errors.username && touched.username && <div>{errors.username}</div>}
<button type="button" onClick={() => validateField("username")}>
Validate Username</button>
<button
type="button"
onClick={async () => {
const result = await validateForm();
console.log(result);
}}
>
Validate All Fields</button>
<button type="submit">Submit</button>
</Form>
)}
</Formik>
</div>
);
In this example, the validateUsername function checks the username field. The form includes a button that calls validateField to validate the username. Additionally, we can validate all fields at once using the validateForm function, which returns a promise that resolves to any errors encountered.
The first video titled "Easy form handling in React using Formik!" provides a practical overview of using Formik for form management, showcasing its capabilities.
The second video "React Formik Tutorial with Yup (React Form Validation)" dives deeper into form validation techniques using Yup alongside Formik.
Conclusion
In summary, Formik simplifies the process of adding field-level validation and offers the ability to manually trigger validation in React forms. This not only enhances user experience but also improves the overall reliability of form handling.