Enhancing Backend Development with Spring & Kotlin Techniques
Written on
Chapter 1: Introduction to Backend Development
In our previous discussion on developing a Spring Boot backend using Kotlin, we covered connecting to a MySQL database and managing data effectively. Today, we aim to delve deeper into the creation of more sophisticated applications by employing specialized methods.
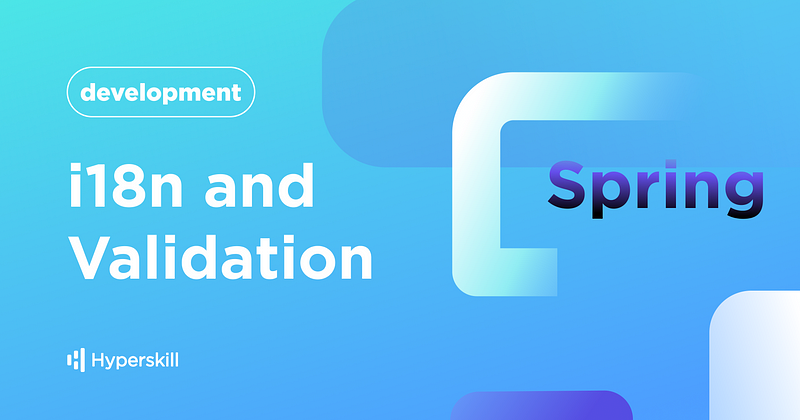
Creating a backend system goes beyond simply selecting appropriate tools and programming languages. It is crucial to integrate strong internationalization (i18n) and validation features to ensure the application can accommodate various inputs and languages. Spring and Kotlin excel in this area, providing developers with a variety of resources and methodologies for implementing these features effectively.
This article will examine different validation and i18n techniques applicable to a Spring and Kotlin backend system. We will cover how to apply validation through Spring’s validation framework and Kotlin's data classes. Additionally, we will discuss how to implement i18n using Spring's message source alongside Kotlin's string templates. By the conclusion of this article, you will have a comprehensive understanding of how to construct a robust backend system utilizing Spring and Kotlin.
As a part of Hyperskill, I encourage you to explore related topics on the platform for a deeper grasp of today’s material. Hyperskill offers various tracks across multiple languages and technologies, not limited to Kotlin and Spring.
Section 1.1: Understanding Internationalization (i18n)
Internationalization (i18n) refers to the design and development of an application in a way that allows for easy adaptation to various languages and regions without altering the fundamental code. This process involves decoupling the application’s user interface and content from its source code, facilitating straightforward translation and localization.
Section 1.2: Implementing i18n in Spring Boot
To incorporate i18n into a Spring Boot application, you need to create message property files for different languages. These files consist of key-value pairs, where each key serves as a unique identifier for a message, and the corresponding value is the translated message in a specified language.
Utilizing i18n messages in your application requires the use of Spring’s ResourceBundleMessageSource. This class manages the loading of message property files, while the LocaleResolver interface identifies the user’s language preference. Below is an example of a configuration class that creates the necessary objects: LocaleResolver and MessageSource.
@Configuration
class MyAppConfig {
@Bean
fun localeResolver(): LocaleResolver {
val localeResolver = AcceptHeaderLocaleResolver()
localeResolver.defaultLocale = Locale.ENGLISH
return localeResolver
}
@Bean("messageSource")
fun messageSource(): MessageSource {
val messageSource = ResourceBundleMessageSource()
messageSource.setBasenames("language/messages")
messageSource.setDefaultEncoding("UTF-8")
return messageSource
}
}
Additionally, you must create locale-specific files in the .properties format. For instance, you would create files with appropriate suffixes for all locales you wish to support:
resources/language/messages.properties:label.delete=User successfully deleted!
resources/language/messages_de.properties:label.delete=Benutzer erfolgreich gelöscht!
Here’s how ResourceBundleMessageSource can be utilized within a Spring Boot controller. While we can enhance our application by adding more logic—such as handling exceptions for non-existent locales or encapsulating message retrieval in a separate function—these details will not be covered in this guide.
@RestController
@RequestMapping("/user")
class UserController(
private val userService: UserService,
private val userMapper: UserMapper,
private val messageSource: ResourceBundleMessageSource,
) {
@DeleteMapping("/{id}")
fun deleteUser(@PathVariable id: Long): DeleteResponse {
userService.deleteUser(id)
val locale = LocaleContextHolder.getLocale()
val msg = messageSource.getMessage("label.delete", null, locale)
return DeleteResponse(msg)
}
}
Request Example:
DELETE localhost:8080/user/5
Accept-Language: de
Response Example:
{
"message": "Benutzer erfolgreich gelöscht!"
}
Request Example:
DELETE localhost:8080/user/6
Accept-Language: en
Response Example:
{
"message": "User successfully deleted!"
}
In this scenario, we inject ResourceBundleMessageSource into our controller, enabling us to retrieve messages based on the user's language preference.
Section 1.3: Best Practices for i18n
When integrating i18n into your Spring Boot application, following best practices is essential for maintaining and scaling your code. Here are key recommendations:
- Maintain a separate properties file for each language to keep translations organized.
- Utilize keys instead of hard-coded strings within your code to simplify updates and maintenance of translations.
- Implement placeholders within message property files to accommodate dynamic content, such as user names or dates.
Chapter 2: The Importance of Validation
Validation is a vital component of any software application, ensuring the accuracy, completeness, and consistency of user input data. It involves verifying user input against predefined rules and constraints, such as confirming that an email address is valid or that a user's age falls within an acceptable range.
In backend systems, validation is crucial for ensuring that processed data adheres to the business rules and requirements of the application. Without appropriate validation, a system may be susceptible to errors, inconsistencies, and even security vulnerabilities.
Section 2.1: Leveraging Spring Validation
Spring Validation is a framework within the Spring ecosystem designed to allow developers to establish and enforce validation rules for user input data. It is based on the Java Bean Validation (JSR-380) standard and provides a set of annotations to define validation constraints on Java objects.
Spring Validation is a powerful resource for guaranteeing the accuracy and completeness of user input in a Spring-based backend. Developers can specify validation rules for individual fields as well as for the overall object. For instance, constraints can be defined for maximum string length, minimum and maximum numeric values, or whether a field is mandatory.
Integrating Spring Validation into a Spring application is straightforward—simply add the necessary dependencies and configure a validator object. Once set up, developers can annotate their Java classes and fields with validation constraints, allowing the validator to automatically check user input against these rules.
To implement this feature, include the following dependency in your build.gradle.kts:
implementation("org.springframework.boot:spring-boot-starter-validation")
Implementation Example:
First, define a data class for your data object, User:
class UserRequest(
@field:NotNull(message = "Name cannot be null")
@field:Size(min = 2, max = 50, message = "Name must be between 2 and 50 characters")
val name: String,
@field:NotNull(message = "Email cannot be null")
@field:Email(message = "Email must be valid")
val email: String,
)
Next, create a controller class to handle requests for creating a new user:
@RestController
class UserController(private val userService: UserService) {
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
fun createUser(@Valid @RequestBody user: UserRequest): UserResponse {
return userMapper.asResponse(userService.createUser(userMapper.asEntity(user)))}
@ExceptionHandler(MethodArgumentNotValidException::class)
fun handleValidationException(ex: MethodArgumentNotValidException): ResponseEntity<String> {
val result = ex.bindingResult
val errors = result.fieldErrors
val message = errors.joinToString("n") { it.defaultMessage }
return ResponseEntity.badRequest().body(message)
}
}
In this example, the UserController class has a createUser() method that processes POST requests for creating a new user. The @Valid annotation on the user parameter indicates that the User object should be validated against its defined annotations. Any validation errors trigger a MethodArgumentNotValidException, which we handle in the handleValidationException() method. This method compiles the validation error messages into a response entity with a 400 Bad Request status.
Test the updated endpoint with invalid data:
POST localhost:8080/user
Content-Type: application/json
{
"name": "n",
"email": "[email protected]"
}
The application will return a 400 HTTP code with the following message:
Name must be between 2 and 50 characters
Email must be valid
Section 2.2: Conclusion on Validation
In summary, Spring Validation serves as an essential mechanism for ensuring the integrity and completeness of user input data in Spring-based backend systems. Through the use of validation annotations, developers can effortlessly establish and enforce validation rules for individual fields and entire objects, ensuring their applications can confidently handle user input.
Moreover, by integrating internationalization (i18n) into your validation messages, a more user-friendly experience can be provided for users across the globe. Utilizing Spring’s MessageSource and LocaleResolver allows you to define validation messages in multiple languages and serve the appropriate message based on the user's language preference.
Together, Spring Validation and i18n contribute to the development of robust and user-friendly backend systems capable of managing a diverse array of user inputs from around the world. So, when constructing a Spring-based backend system, be sure to incorporate both Spring Validation and i18n into your development strategy!
If you seek to deepen your understanding of Spring Validation and avoid common pitfalls, consider enrolling in a course on Spring through our educational platform, Hyperskill!
Our primary content provider, JetBrains, offers interactive and comprehensive tracks on Spring and Kotlin, as well as other programming languages and technologies.
For further learning, explore the following topics:
- Bean Validation
- Spring Beans
- Spring Actuator
Additionally, if you're interested in studying Kotlin, check out our tracks on Kotlin Basics and Kotlin Developer!
I hope this article proves useful. Should you have any inquiries or feedback, feel free to share in the comments section below.
Thank you for reading, and happy coding!
Chapter 3: Recommended Resources
To enhance your learning experience, consider these video tutorials:
Discover the best tutorials and books for mastering Spring Framework and JAVA Backend Development.
Learn about Full Stack Development using Java Spring Boot, React, and MongoDB.