Understanding Java's Memory Management: Generational Garbage Collection
Written on
Chapter 1: An Introduction to Generational Garbage Collection
If you're seeking a straightforward explanation of the young generation, old generation, and permanent generation within the context of Java, you've come to the right place. Although these terms may seem more applicable to people than to software concepts, understanding them is crucial for grasping Java's garbage collection.
Java Garbage Collection Overview
In programming languages like C, developers must meticulously manage memory by explicitly allocating and deallocating it. Conversely, Java automates this process with its garbage collection system, allowing developers to focus less on manual memory management. Essentially, objects in Java are stored in a heap, also referred to as heap memory. The garbage collection algorithm scans this heap, marking objects still in use by the Java Virtual Machine (JVM) and subsequently reclaiming memory from those that are not marked.
The specifics of this algorithm warrant a deeper discussion, but it is vital to understand that the heap is segmented into smaller parts known as generations.
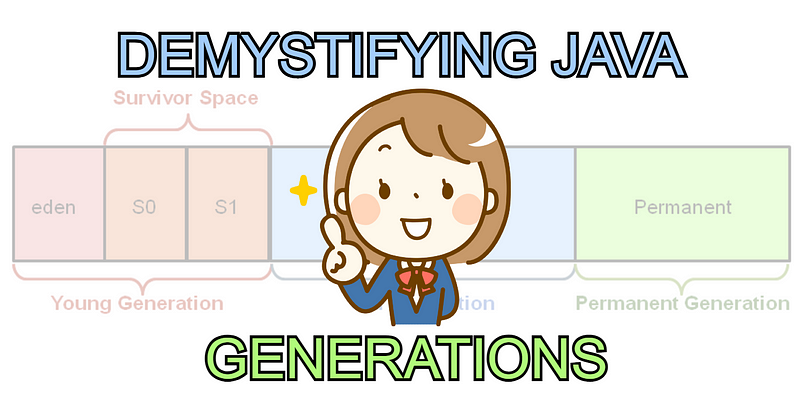
The diagram above introduces the terms “young generation,” “old generation,” and “permanent generation.” Although these concepts may seem complex at first glance, they are quite intuitive once broken down.
The Young Generation
At a high level, the young generation is where all new objects are initially allocated. When objects are created in Java, they reside in a specific area known as the eden space. As the eden space fills up, a minor garbage collection event is triggered. During this event, the marking algorithm identifies which objects are referenced and marks them accordingly, while unreferenced objects are cleared out.
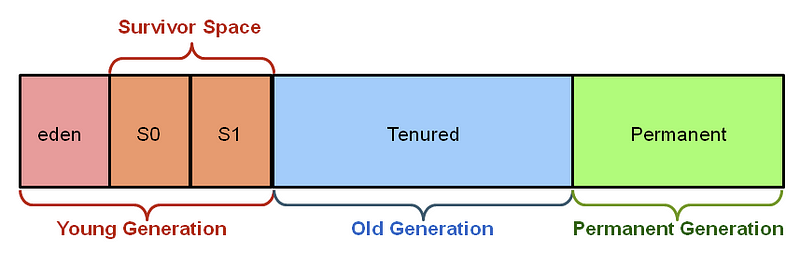
Objects that survive this marking process move to a section of the young generation called S0 of the survivor space, which is split into two parts: S0 and S1. When the eden space fills again, a new cycle begins, resulting in previously marked objects from both the eden space and S0 moving to S1. The diagram below illustrates this process.
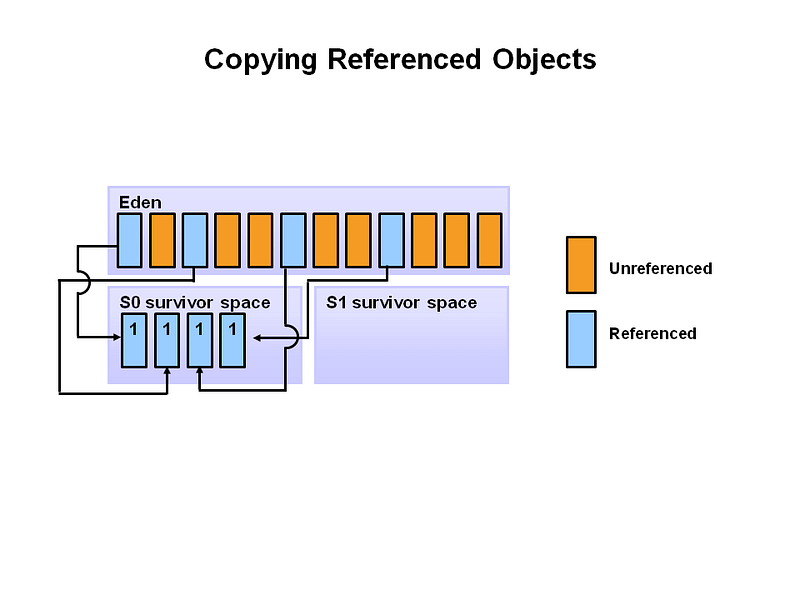
It is important to note that objects reaching the survivor space are assigned an age counter. This counter is used by the algorithm to determine if an object qualifies for promotion to the old generation.
As the process continues, when the eden space fills up again, the roles of the from and to survivor spaces are switched, leading to a more complex but systematic management of memory.
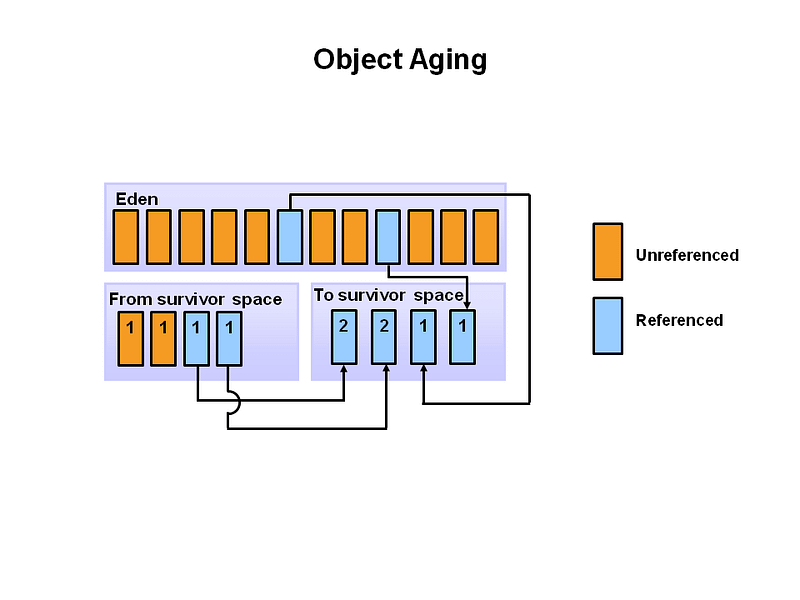
The key takeaway here is that all new objects start in the eden space and may eventually be promoted to the survivor space after surviving garbage collection cycles.
The Old Generation
The old generation is essentially where long-lived objects reside. If objects surpass a certain age threshold after several garbage collection cycles in the young generation, they are promoted to the old generation. When objects are garbage collected from this area, a major garbage collection event occurs.
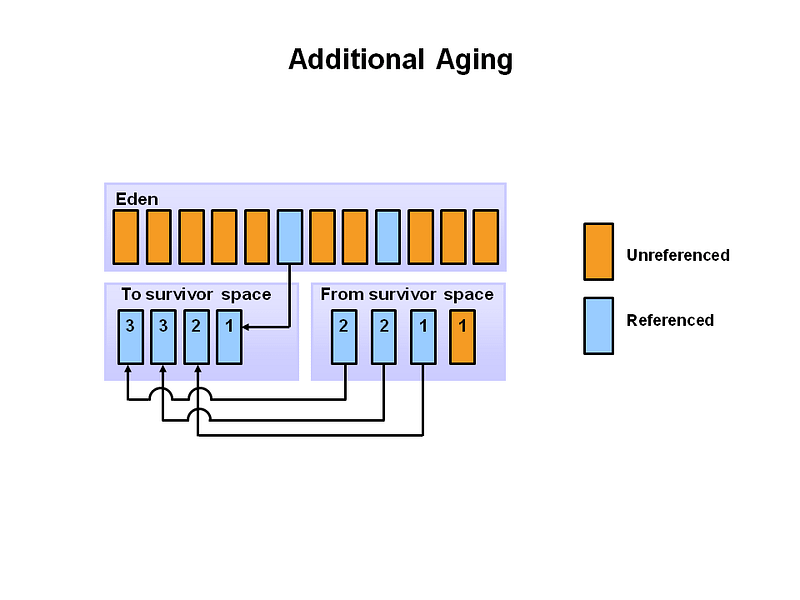
In the diagram above, any surviving objects that reach a hypothetical age threshold—say, eight cycles—are moved to the old generation. This section is often referred to as the tenured generation, and the terms are frequently used interchangeably.
The Permanent Generation
Now, here's an important distinction: the permanent generation is not filled by objects from the old generation. Instead, it is populated by the JVM with metadata representing the application’s classes and methods during runtime. Occasionally, the JVM may clean out the permanent generation, which is referred to as a full garbage collection.
Understanding "Stop the World" Events
A "stop the world" event may sound dramatic, but it simply refers to the suspension of all application threads during a minor or major garbage collection event. During these times, the Java application essentially comes to a halt as garbage collection takes place.
Conclusion
In summary, this article aims to clarify the foundational concepts behind the young generation, old generation, and permanent generation within Java’s automatic garbage collection process. While the intricacies can be complex, familiarizing yourself with these terms and their general functions is beneficial.
Explore the JVM and the intricacies of Java Garbage Collection in this recorded webcast event.
A comprehensive guide for beginners on Java, focusing on Garbage Collection in this tutorial.