How to Effectively Utilize Terraform's toset Function
Written on
Understanding the Terraform toset Function
In this brief guide, we will explore the type conversion function known as toset() in Terraform. We'll clarify its applications, differentiate it from the tolist() function, and provide practical examples of how to implement it within a for_each loop.
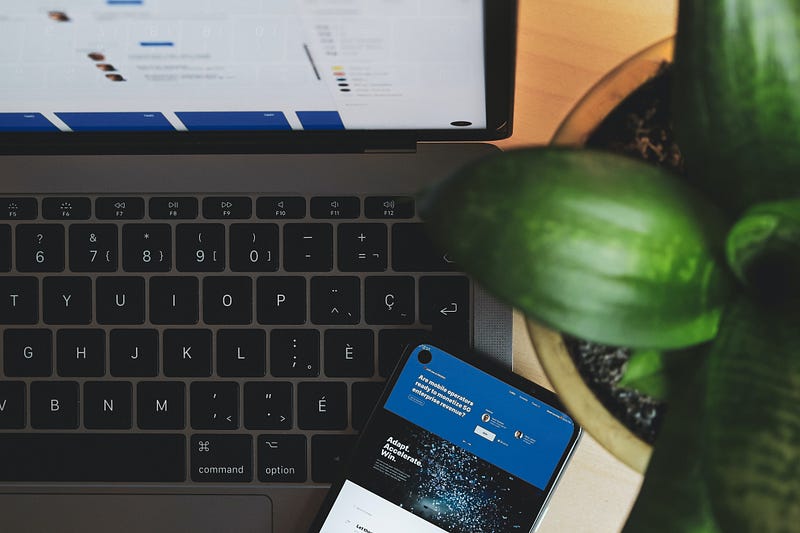
What is the toset Function?
The toset function serves as a type conversion utility in Terraform, transforming its argument into a set value. A set in Terraform is defined as an unordered collection of unique elements. This collection type is particularly useful when you need to manage distinct items, ensuring that each element is unique without concern for order.
Differentiating toset from tolist
The key distinction between toset() and tolist() lies in how they handle values:
- toset() converts the input into a set, which guarantees uniqueness and disregards order.
- tolist() transforms the input into a list, maintaining the sequence of elements.
In Terraform, a list is an ordered collection of elements. This means that the arrangement of items in a list is preserved, allowing for varied types of elements including strings, numbers, or more complex structures.
Using the toset Function — A Practical Example
The syntax for using toset is straightforward: toset(value), where the value is the input you wish to convert to a set. Consider the following example where we convert a list into a set:
variable "my_list" {
type = list(string)
default = ["luke", "yoda", "darth"]
}
locals {
my_set = toset(var.my_list)
}
output "set_output" {
value = local.my_set
}
The output will appear as follows:
Outputs:
set_output = toset([
"luke",
"yoda",
"darth",
])
You can also experiment with the Terraform console to test functions like toset(). For instance, directly supplying values to the toset() function will yield similar results.
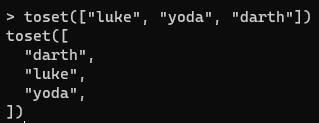
It's important to note that since all elements in a Terraform set must be of the same type, if you provide mixed types (e.g., strings and numbers), they will be converted to the most general type. Additionally, any duplicates in the input will be eliminated, as sets do not allow for repeated values. The original order of the list will also be lost in the conversion process.
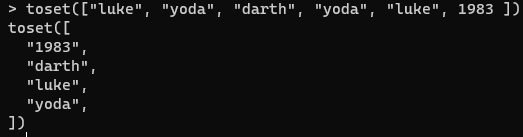
Utilizing toset with for_each
The for_each construct in Terraform is a powerful tool for iterating over a map or set of values to create multiple instances of a resource or module. When you wish to utilize for_each with toset(), you must first convert your data into a set. Here’s an example:
variable "my_list" {
type = list(string)
default = ["luke", "yoda", "darth"]
}
resource "example_resource" "my_resource" {
for_each = toset(var.my_list)
name = each.value
}
output "character_names" {
value = example_resource.my_resource[*].name
}
Upon executing terraform apply, the output will display:
Outputs:
character_names = [
"luke",
"yoda",
"darth",
]
Key Takeaways
In Terraform, type conversion functions are essential for transforming values between different data types. The toset() function is particularly useful for converting values into a set, ensuring both uniqueness and an unordered structure.
For additional details about the toset() function, you can visit the official documentation pages:
Feel free to explore my other articles on Terraform as well!
Best regards! 🌟
Jack Roper, passionate about Azure, Azure DevOps, Terraform, Kubernetes, and Cloud technology! I hope this guide was helpful and enjoyable for you. I love sharing technical insights and experiences through writing.
Originally published at spacelift.io.